atom
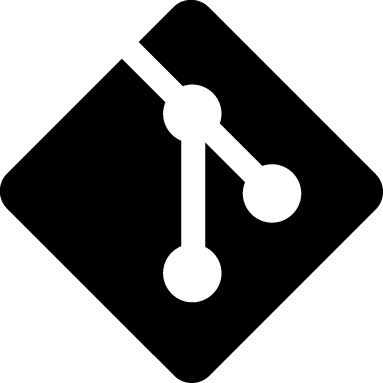
Vars | |
damage_hitsound | Sound effect played when hit |
---|---|
health_current | Current health for health processing. Use get_current_health() , damage_health() , or restore_health() for general health references. |
health_dead | Boolean. Whether or not the atom is dead. Toggled by death state changes in standardized health and provided as a simple way to check for death without additional proc call overhead from is_alive() . |
health_max | Maximum health for simple health processing. Use get_max_health() or set_max_health() to reference/modify. |
health_min_damage | Minimum damage required to actually affect health in can_damage_health() . |
health_resistances | LAZY List of damage type resistance or weakness multipliers, decimal form. Only applied to health reduction. Use set_damage_resistance() , remove_damage_resistance() , and get_damage_resistance() to reference/modify. |
tf_offset_x | The atom's base transform scale for horizontal offset. |
tf_offset_y | The atom's base transform scale for vertical offset. |
tf_rotation | The atom's base transform scale for rotation. |
tf_scale_x | The atom's base transform scale for width. |
tf_scale_y | The atom's base transform scale for height. |
Procs | |
Adjacent | Adjacency proc for determining touch range |
AltClick | Called when a mob alt+clicks the atom. By default, this creates and populates the Turf panel, displaying all objects on the atom's turf. |
Beam | This is what you use to start a beam. Example: origin.Beam(target, args). Store the return of this proc if you don't set maxdist or time, you need it to delete the beam. |
ClearTransform | Clear the atom's tf_* variables and the current transform state. |
CtrlAltClick | Called when a mob ctrl+alt+clicks on the atom. |
CtrlClick | Called when a mob ctrl+clicks on the atom. By default, this starts pulling a movable atom if adjacent. |
CtrlShiftClick | Called when a mob ctrl+shift+clicks on the atom. |
Entered | Movement Handling |
Exited | Exited Handling |
IsCoil | True when this atom can be used as a cable coil. |
IsCrowbar | True when this atom can be used as a crowbar. |
IsHatchet | True when this atom can be used as a hatchet. |
IsMultitool | True when this atom can be used as a multitool. |
IsScrewdriver | True when this atom can be used as a screwdriver. |
IsWelder | True when this atom can be used as a Welder. |
IsWirecutter | True when this atom can be used as a wirecutter. |
IsWrench | True when this atom can be used as a wrench. |
SetName | Name Set Handling |
SetTransform | Sets the atom's tf_* variables and the current transform state, also applying others if supplied. |
ShiftClick | Called when a mob shift+clicks on the atom. By default, this calls the examine proc chain. |
attack_ai | Called when an AI mob clicks on an atom. |
attack_animal | Called when a simple_animal mob clicks on the atom with an 'empty hand.' |
attack_generic | Generic attack and damage proc, called on the attacked atom. |
attack_ghost | Called when a ghost mob clicks on an atom. |
can_damage_health | Checks if the atom's health can be damaged.
Should be called before damage_health() in most cases.
Returns null if health is not in use. |
can_restore_health | Checks if the atom's health can be restored.
Should be called before restore_health() in most cases.
Returns null if health is not in use.
NOTE: Does not include a check for death state by default, to allow repairing/healing atoms back to life. |
damage_health | Damage's the atom's health by the given value. Returns TRUE if the damage resulted in a death state change.
Resistance and weakness modifiers are applied here. |
examine_damage_state | Handles sending damage state to users on examine() .
Overrideable to allow for different messages, or restricting when the messages can or cannot appear. |
get_alarm_z | Assisting procs |
get_current_health | Retrieves the atom's current health, or null if not using health |
get_damage_percentage | Retrieves the atom's current damage as a percentage where 100% is 100 .
If use_raw_values is TRUE , uses the raw var values instead of the get_* proc results. |
get_damage_resistance | Fetches the atom's current resistance value for the given damage type. |
get_damage_value | Retrieves the atom's current damage, or null if not using health.
If use_raw_values is TRUE , uses the raw var values instead of the get_* proc results. |
get_max_health | Retrieves the atom's maximum health. |
health_damaged | Whether or not the atom's health is damaged. |
is_damage_immune | Determines whether or not the atom has full immunity to the given damage type. |
kill_health | Immediately sets health to 0 then updates death_state , bypassing all other checks and processes. |
mod_health | Health modification for the health system. Applies health_mod directly to simple_health via addition and calls handle_death_change as needed.
Has no pre-modification checks, you should be using damage_health() or restore_health() instead of this.
skip_death_state_change will skip calling handle_death_change() when applicable. Used for when the originally calling proc needs handle it in a unique way.
Returns TRUE if the death state changes, null if the atom is not using health, FALSE otherwise. |
on_death | Proc called when the atom transitions from alive to dead. |
on_revive | Proc called when the atom transitions from dead to alive. |
post_health_change | Proc called after any health changes made by the system |
remove_damage_resistance | Removes the atom's resistance/weakness to the given damage type. |
restore_health | Restore's the atom's health by the given value. Returns TRUE if the restoration resulted in a death state change. |
revive_health | Returns health to full, resetting the death state as well. |
set_damage_resistance | Sets the atom's resistance/weakness to the given damage type.
Value should be a multiplier that is applied against damage. Values below 1 are a resistance, above 1 are a weakness.
Value of 0 is considered immunity. |
set_dir | Called to set the atom's dir and used to add behaviour to dir-changes |
set_health | Sets health_current to the new value, clamped between 0 and health_max .
Has no pre-modification checks.
Returns TRUE if the death state changes, null if the atom is not using health, FALSE otherwise. |
set_invisibility | Invisibility Set Handling |
set_max_health | Sets the atoms maximum health to the new value.
If set_current_health is TRUE , also sets the current health to the new value. |
singuloCanEat | Nar-Sie Act/Pull |
Var Details
damage_hitsound
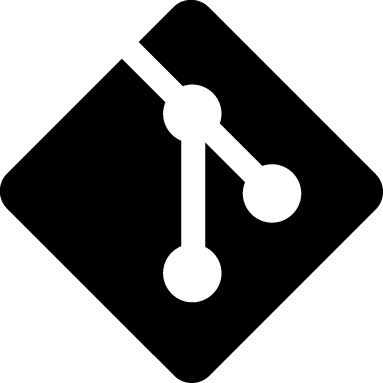
Sound effect played when hit
health_current
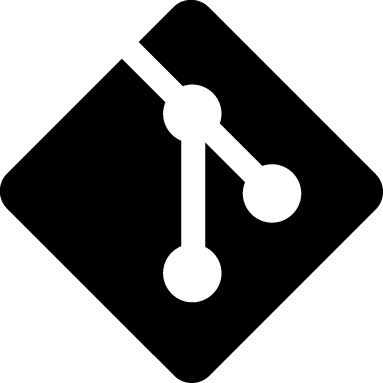
Current health for health processing. Use get_current_health()
, damage_health()
, or restore_health()
for general health references.
health_dead
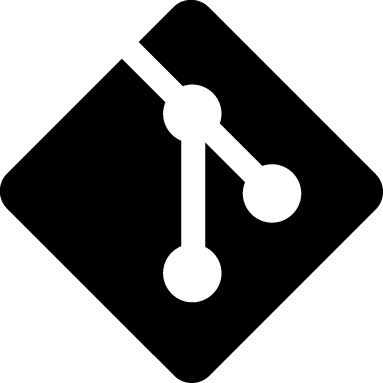
Boolean. Whether or not the atom is dead. Toggled by death state changes in standardized health and provided as a simple way to check for death without additional proc call overhead from is_alive()
.
health_max
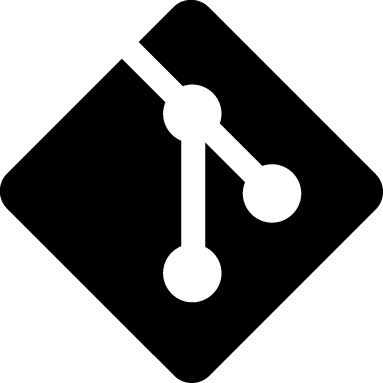
Maximum health for simple health processing. Use get_max_health()
or set_max_health()
to reference/modify.
health_min_damage
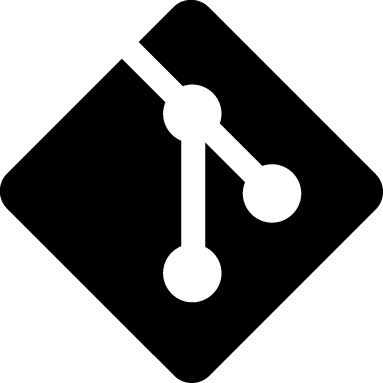
Minimum damage required to actually affect health in can_damage_health()
.
health_resistances
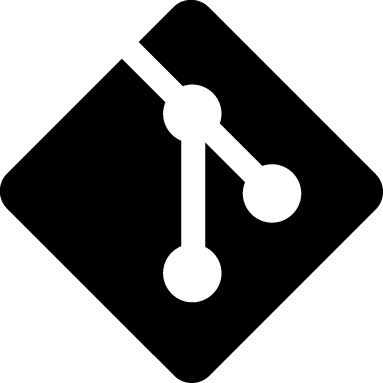
LAZY List of damage type resistance or weakness multipliers, decimal form. Only applied to health reduction. Use set_damage_resistance()
, remove_damage_resistance()
, and get_damage_resistance()
to reference/modify.
Index should be one of the DAMAGE_
flags.
Value should be a multiplier that is applied against damage. Values below 1 are a resistance, above 1 are a weakness.
Value of 0
is considered immunity.
tf_offset_x
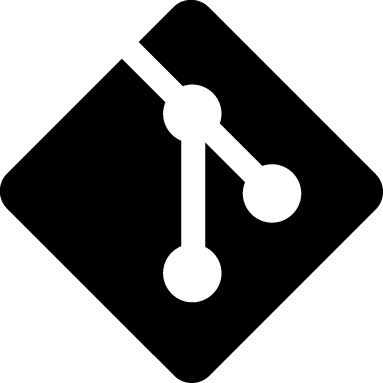
The atom's base transform scale for horizontal offset.
tf_offset_y
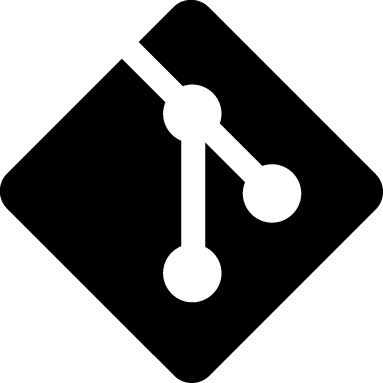
The atom's base transform scale for vertical offset.
tf_rotation
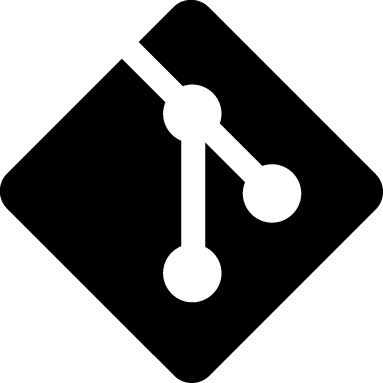
The atom's base transform scale for rotation.
tf_scale_x
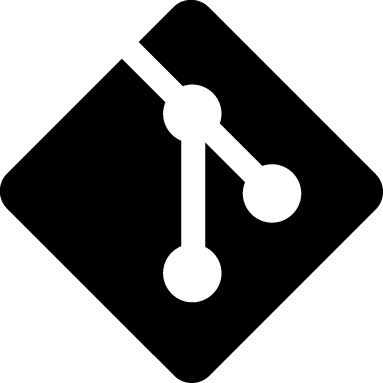
The atom's base transform scale for width.
tf_scale_y
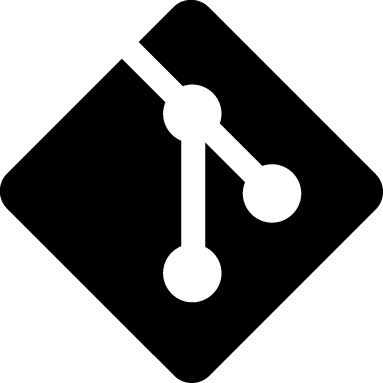
The atom's base transform scale for height.
Proc Details
Adjacent
Adjacency proc for determining touch range
This is mostly to determine if a user can enter a square for the purposes of touching something. Examples include reaching a square diagonally or reaching something on the other side of a glass window.
This is calculated by looking for border items, or in the case of clicking diagonally from yourself, dense items. This proc will NOT notice if you are trying to attack a window on the other side of a dense object in its turf. There is a window helper for that.
Note that in all cases the neighbor is handled simply; this is usually the user's mob, in which case it is up to you to check that the mob is not inside of something
AltClick
Called when a mob alt+clicks the atom. By default, this creates and populates the Turf panel, displaying all objects on the atom's turf.
Parameters:
user
- The mob that clicked on the atom.
Returns boolean - Whether or not the action was handled.
Beam
This is what you use to start a beam. Example: origin.Beam(target, args). Store the return of this proc if you don't set maxdist or time, you need it to delete the beam.
Unless you're making a custom beam effect (see the beam_type argument), you won't actually have to mess with any other procs. Make sure you store the return of this Proc, you'll need it to kill the beam. Arguments:
- BeamTarget: Where you're beaming from. Where do you get origin? You didn't read the docs, fuck you.
- icon_state: What the beam's icon_state is. The datum effect isn't the ebeam object, it doesn't hold any icon and isn't type dependent.
- icon: What the beam's icon file is. Don't change this, man. All beam icons should be in beam.dmi anyways.
- maxdistance: how far the beam will go before stopping itself. Used mainly for two things: preventing lag if the beam may go in that direction and setting a range to abilities that use beams.
- beam_type: The type of your custom beam. This is for adding other wacky stuff for your beam only. Most likely, you won't (and shouldn't) change it.
ClearTransform
Clear the atom's tf_* variables and the current transform state.
CtrlAltClick
Called when a mob ctrl+alt+clicks on the atom.
Parameters:
user
- The mob that clicked on the atom.
Returns boolean - Whather or not the interaction was handled.
CtrlClick
Called when a mob ctrl+clicks on the atom. By default, this starts pulling a movable atom if adjacent.
Parameters:
user
- The mob that clicked on the atom.
Returns boolean - Whether or not the action was handled.
CtrlShiftClick
Called when a mob ctrl+shift+clicks on the atom.
Parameters:
user
- The mob that clicked on the atom.
Entered
Movement Handling
Exited
Exited Handling
IsCoil
True when this atom can be used as a cable coil.
IsCrowbar
True when this atom can be used as a crowbar.
IsHatchet
True when this atom can be used as a hatchet.
IsMultitool
True when this atom can be used as a multitool.
IsScrewdriver
True when this atom can be used as a screwdriver.
IsWelder
True when this atom can be used as a Welder.
IsWirecutter
True when this atom can be used as a wirecutter.
IsWrench
True when this atom can be used as a wrench.
SetName
Name Set Handling
SetTransform
Sets the atom's tf_* variables and the current transform state, also applying others if supplied.
ShiftClick
Called when a mob shift+clicks on the atom. By default, this calls the examine proc chain.
Parameters:
user
- The mob that clicked on the atom.
attack_ai
Called when an AI mob clicks on an atom.
Parameters:
user
- The mob clicking on the atom.
attack_animal
Called when a simple_animal
mob clicks on the atom with an 'empty hand.'
Parameters:
user
- The mob clicking on the atom.
attack_generic
Generic attack and damage proc, called on the attacked atom.
Parameters:
user
- The attacking mob.damage
(int) - The damage value.attack_verb
(string) - The verb/string used for attack messages.wallbreaker
(boolean) - Whether or not the attack is considered a 'wallbreaker' attack - I.e., hulk.damtype
(string, one ofDAMAGE_*
) - The attack's damage type.armorcheck
(string) - TODO: Unused. Remove.dam_flags
(bitfield, any ofDAMAGE_FLAG_*
) - Damage flags associated with the attack.
Returns boolean. TODO: Return value is unused. Remove.
attack_ghost
Called when a ghost mob clicks on an atom.
Parameters:
user
- The mob clicking on the atom.
can_damage_health
Checks if the atom's health can be damaged.
Should be called before damage_health()
in most cases.
Returns null
if health is not in use.
can_restore_health
Checks if the atom's health can be restored.
Should be called before restore_health()
in most cases.
Returns null
if health is not in use.
NOTE: Does not include a check for death state by default, to allow repairing/healing atoms back to life.
damage_health
Damage's the atom's health by the given value. Returns TRUE
if the damage resulted in a death state change.
Resistance and weakness modifiers are applied here.
damage_type
should be one of theDAMAGE_*
damage types, ornull
. Defining a damage type is preferable over not.damage_flags
is a bitfield ofDAMAGE_FLAG_*
values.severity
should be a passthrough ofseverity
fromex_act()
andemp_act()
forDAMAGE_EXPLODE
andDAMAGE_EMP
types respectively.skip_can_damage_check
(boolean) - IfTRUE
skips checkingcan_damage_health()
. Intended for cases where this was already checked.
examine_damage_state
Handles sending damage state to users on examine()
.
Overrideable to allow for different messages, or restricting when the messages can or cannot appear.
get_alarm_z
Assisting procs
get_current_health
Retrieves the atom's current health, or null
if not using health
get_damage_percentage
Retrieves the atom's current damage as a percentage where 100%
is 100
.
If use_raw_values
is TRUE
, uses the raw var values instead of the get_*
proc results.
get_damage_resistance
Fetches the atom's current resistance value for the given damage type.
get_damage_value
Retrieves the atom's current damage, or null
if not using health.
If use_raw_values
is TRUE
, uses the raw var values instead of the get_*
proc results.
get_max_health
Retrieves the atom's maximum health.
health_damaged
Whether or not the atom's health is damaged.
is_damage_immune
Determines whether or not the atom has full immunity to the given damage type.
kill_health
Immediately sets health to 0
then updates death_state
, bypassing all other checks and processes.
mod_health
Health modification for the health system. Applies health_mod
directly to simple_health
via addition and calls handle_death_change
as needed.
Has no pre-modification checks, you should be using damage_health()
or restore_health()
instead of this.
skip_death_state_change
will skip calling handle_death_change()
when applicable. Used for when the originally calling proc needs handle it in a unique way.
Returns TRUE
if the death state changes, null
if the atom is not using health, FALSE
otherwise.
on_death
Proc called when the atom transitions from alive to dead.
on_revive
Proc called when the atom transitions from dead to alive.
post_health_change
Proc called after any health changes made by the system
remove_damage_resistance
Removes the atom's resistance/weakness to the given damage type.
restore_health
Restore's the atom's health by the given value. Returns TRUE
if the restoration resulted in a death state change.
revive_health
Returns health to full, resetting the death state as well.
set_damage_resistance
Sets the atom's resistance/weakness to the given damage type.
Value should be a multiplier that is applied against damage. Values below 1 are a resistance, above 1 are a weakness.
Value of 0
is considered immunity.
set_dir
Called to set the atom's dir and used to add behaviour to dir-changes
set_health
Sets health_current
to the new value, clamped between 0
and health_max
.
Has no pre-modification checks.
Returns TRUE
if the death state changes, null
if the atom is not using health, FALSE
otherwise.
set_invisibility
Invisibility Set Handling
set_max_health
Sets the atoms maximum health to the new value.
If set_current_health
is TRUE
, also sets the current health to the new value.
singuloCanEat
Nar-Sie Act/Pull